Understanding the Loop: A Simple Explanation of (let i = 0; i < 5; i++)
If you’re new to programming or JavaScript, loops can seem a bit confusing at first. But don’t worry—they’re actually one of the most powerful and straightforward tools in coding! Let’s break down a common loop example step by step and understand how it works.
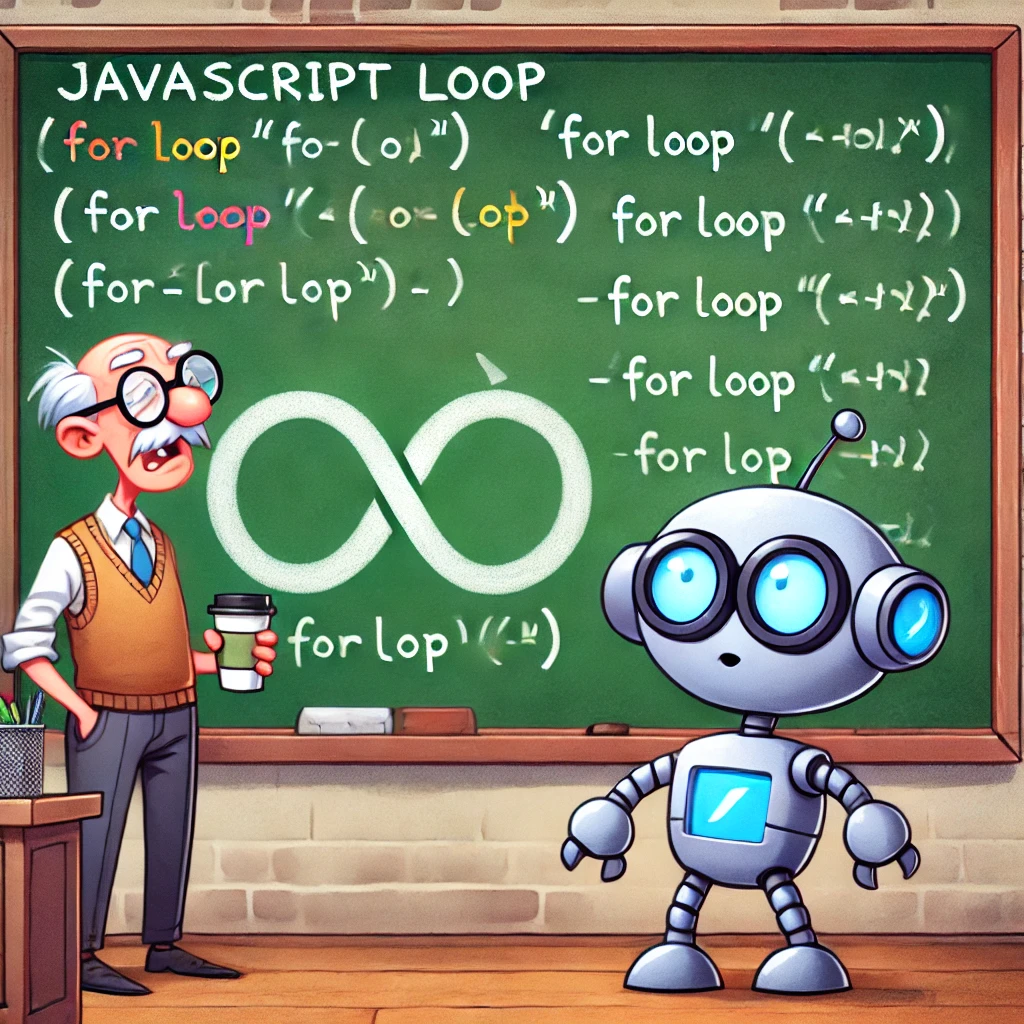
The Code in Question
Here’s the code we’ll be exploring:
for (let i = 0; i < 5; i++) {
setTimeout(function() {
console.log(i); // Outputs 0, 1, 2, 3, 4
}, 1000);
}
At first glance, this might look a little intimidating, but let’s simplify it.
What is a Loop?
A loop is a way to repeat a block of code multiple times. Instead of writing the same code over and over, you can use a loop to do the work for you. In this case, the loop runs a piece of code 5 times.
Breaking Down the Loop
Let’s dissect the loop line by line:
1. for (let i = 0; i < 5; i++)
This is the loop declaration. It has three parts:
- Initialization (
let i = 0
): This sets up a variablei
and gives it an initial value of0
. Think ofi
as a counter that starts at 0. - Condition (
i < 5
): This tells the loop when to stop. As long asi
is less than 5, the loop will keep running. - Increment (
i++
): After each loop iteration,i
increases by 1 (i++
is shorthand fori = i + 1
).
2. setTimeout(function() { ... }, 1000)
This is the code inside the loop. setTimeout
is a function that delays the execution of code. In this case, it waits 1000 milliseconds (1 second) before running the code inside it.
3. console.log(i)
This prints the value of i
to the console. It’s what we’ll see as the output.
How the Loop Works
Let’s walk through what happens step by step:
- First Iteration (
i = 0
):
- The loop starts with
i = 0
. setTimeout
schedulesconsole.log(i)
to run after 1 second.- The loop increments
i
to1
.
- Second Iteration (
i = 1
):
- The loop checks the condition (
i < 5
). Since1 < 5
, it continues. setTimeout
schedules anotherconsole.log(i)
to run after 1 second.- The loop increments
i
to2
.
- Third Iteration (
i = 2
):
- The loop checks the condition (
2 < 5
) and continues. setTimeout
schedules anotherconsole.log(i)
.- The loop increments
i
to3
.
- Fourth Iteration (
i = 3
):
- The loop checks the condition (
3 < 5
) and continues. setTimeout
schedules anotherconsole.log(i)
.- The loop increments
i
to4
.
- Fifth Iteration (
i = 4
):
- The loop checks the condition (
4 < 5
) and continues. setTimeout
schedules anotherconsole.log(i)
.- The loop increments
i
to5
.
- Loop Ends (
i = 5
):
- The loop checks the condition (
5 < 5
). Since this is false, the loop stops.
What Happens After 1 Second?
After 1 second, all the scheduled console.log(i)
statements execute. Because we used let
to declare i
, each iteration of the loop has its own i
value. This is called block scoping.
Here’s what gets printed:
0
1
2
3
4
Why Does This Happen?
- Block Scoping with
let
: When you uselet
, the variablei
is scoped to the block (inside the loop). Each iteration gets its owni
, so the value doesn’t get overwritten. - Delayed Execution with
setTimeout
: Even though the loop runs quickly, thesetTimeout
function delays theconsole.log(i)
by 1 second. By the time the logs execute, the loop has already finished, but the correct values are preserved.
What If We Used var
Instead of let
?
If you replace let
with var
, the output would be different:
for (var i = 0; i < 5; i++) {
setTimeout(function() {
console.log(i); // Outputs 5, five times
}, 1000);
}
This happens because var
is function-scoped, not block-scoped. By the time the setTimeout
functions run, the loop has already finished, and i
has reached 5
.
Key Takeaways
- Loops are a way to repeat code without writing it multiple times.
let
ensures that each iteration of the loop has its own variable, thanks to block scoping.setTimeout
delays the execution of code, which can lead to interesting behavior when combined with loops.- Always use
let
orconst
instead ofvar
to avoid unexpected bugs.
Try It Yourself!
Copy the code into your browser’s console or a code editor and run it. Experiment with changing the loop condition, delay time, or using var
instead of let
to see how the output changes.
Conclusion
Loops are a fundamental concept in programming, and understanding how they work is crucial for writing efficient code. By breaking down the example step by step, we’ve seen how a simple loop can produce powerful results. Keep practicing, and soon loops will become second nature to you!
Happy coding! 🚀
You must be logged in to post a comment.