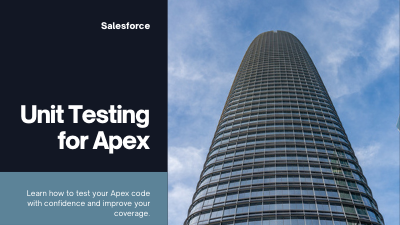
Imagine you’re preparing a small party and you need to make sure everything is set before your guests arrive. In the Salesforce world, that’s like setting up a unit test for our BatchMaskAction
class. We want to ensure our batch job tidies up our Actions__c
records just as we planned.
Here’s how we’ll do it:
- Prep the Place: We’ll create a few
Actions__c
records as our “party setup”. Think of these as decorations and snacks. We need these to see if our batch job does its cleaning magic right. - Start the Party: Next, we’ll kick things off with
Test.startTest()
, invite ourBatchMaskAction
job to the scene withDatabase.executeBatch(batchJob)
, and wrap up withTest.stopTest()
. This makes sure our job runs during the party and not after everyone’s gone home. - Check the Cleanup: After the batch job has done its thing, we’ll look around to see if our
Actions__c
records are as clean as we expected. EachDescription__c
andResolution_Comments__c
should be shiny and new, just like we hoped.
Here’s a simplified script that gets this done:
@isTest
private class TestBatchMaskAction {
static testMethod void makeSureOurPartyRocks() {
// Prep the place with some Actions__c records
List<Actions__c> prepList = new List<Actions__c>();
for(Integer i = 0; i < 3; i++) {
prepList.add(new Actions__c(
Description__c = 'Before Party Desc ' + i,
Resolution_Comments__c = 'Before Party Comments ' + i
));
}
insert prepList;
// Start the party and run the cleanup crew
Test.startTest();
BatchMaskAction batchJob = new BatchMaskAction();
Database.executeBatch(batchJob);
Test.stopTest();
// Check the cleanup
List<Actions__c> aftermathCheck = [SELECT Id, Description__c, Resolution_Comments__c FROM Actions__c];
for(Actions__c a : aftermathCheck) {
System.assert(a.Description__c.startsWith('Description '), 'Uh oh, the description didn't get cleaned up.');
System.assert(a.Resolution_Comments__c.startsWith('Resolution Comments '), 'Oops, the comments are still messy.');
}
}
}
And there you have it—a friendly and simplified way to ensure our BatchMaskAction
does its job correctly, keeping our Actions__c
records neat and tidy.