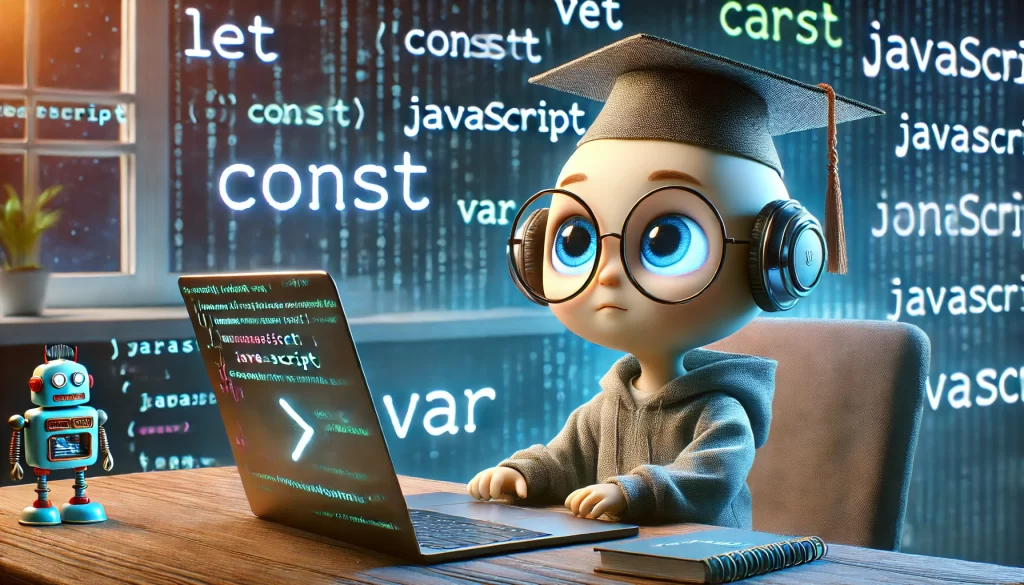
Why You Should Stop Using var
in JavaScript and Switch to let
and const
JavaScript has evolved significantly over the years, and with the introduction of ES6 (ECMAScript 2015), developers gained two powerful new ways to declare variables: let
and const
. These modern alternatives to var
offer better control, readability, and predictability in your code. In this post, we’ll explore why you should stop using var
and embrace let
and const
instead.
1. Block Scoping: A Game-Changer
One of the biggest issues with var
is its function scope. Variables declared with var
are scoped to the nearest function, which can lead to unexpected behavior, especially in loops or conditional statements.
Example with var
:
for (var i = 0; i < 5; i++) {
setTimeout(function() {
console.log(i); // Outputs 5, five times
}, 1000);
}
Here, var
causes the variable i
to be shared across the entire function, leading to unintended results.
Example with let
:
for (let i = 0; i < 5; i++) {
setTimeout(function() {
console.log(i); // Outputs 0, 1, 2, 3, 4
}, 1000);
}
With let
, the variable i
is block-scoped, meaning it’s only accessible within the loop. This eliminates confusion and makes your code more predictable.
2. Preventing Accidental Re-declarations
Another problem with var
is that it allows you to re-declare the same variable within the same scope without throwing an error. This can lead to bugs that are hard to trace.
Example with var
:
var x = 10;
var x = 20; // No error, but this can be confusing
console.log(x); // Outputs 20
Example with let
:
let y = 10;
let y = 20; // SyntaxError: Identifier 'y' has already been declared
Using let
prevents accidental re-declarations, making your code safer and easier to debug.
3. Immutable Variables with const
If you need a variable that should never change, const
is the perfect choice. It ensures that the value remains constant, preventing accidental reassignments.
Example with const
:
const PI = 3.14159;
PI = 3.14; // TypeError: Assignment to constant variable
Using const
for values that shouldn’t change (like configuration settings or mathematical constants) makes your code more robust and self-documenting.
4. Avoiding the Pitfalls of Hoisting
Variables declared with var
are hoisted, meaning they’re moved to the top of their scope during compilation. This can lead to confusing behavior, such as accessing a variable before it’s declared.
Example with var
:
console.log(z); // Outputs undefined (no error)
var z = 10;
Example with let
:
console.log(a); // ReferenceError: Cannot access 'a' before initialization
let a = 10;
With let
and const
, variables are hoisted but remain in a temporal dead zone until they’re declared. This prevents access before initialization and reduces bugs.
5. Better Code Readability and Maintainability
Using let
and const
makes your code more explicit and intentional. By choosing the right keyword for each variable, you communicate its purpose clearly:
- Use
let
for variables that will change. - Use
const
for variables that won’t change.
This improves readability and helps other developers (or your future self) understand the code faster.
6. Future-Proofing Your Code
As JavaScript continues to evolve, best practices are shifting toward let
and const
. Modern frameworks like React, Angular, and Vue encourage the use of let
and const
over var
. By adopting these practices now, you’ll ensure your codebase remains up-to-date and compatible with future JavaScript updates.
When Should You Use var
?
In modern JavaScript, there’s almost no reason to use var
. The only exception might be when working with legacy codebases that rely on var
for specific behaviors. However, even in those cases, it’s better to refactor the code to use let
and const
.
Conclusion
The introduction of let
and const
in ES6 has made var
largely obsolete. By switching to let
and const
, you can write cleaner, more predictable, and maintainable code. Here’s a quick summary of when to use each:
- Use
let
for variables that will change. - Use
const
for variables that won’t change. - Avoid
var
unless you’re working with legacy code.
By adopting these modern JavaScript practices, you’ll not only improve your coding skills but also contribute to a more robust and error-free codebase. Happy coding!
FAQs
Q: Can I use let
and const
in all browsers?
A: Yes, let
and const
are supported in all modern browsers. For older browsers like Internet Explorer, you may need to use a transpiler like Babel.
Q: Is const
really immutable?
A: No, const
only prevents reassignment of the variable itself. For objects and arrays, the properties or elements can still be modified.
Q: Should I always use const
by default?
A: Yes, it’s a good practice to start with const
and only switch to let
if you need to reassign the variable.
You must be logged in to post a comment.